I am going to show you what you need to do to create a Python bot of your own by giving you a general recipe that you can extend at your will.
What is a Python Bot
A Python bot is a script that runs 24/7 hours, without needing to sleep or rest, and can perform any kind of automated task that is doable via a web browser and with an internet connection.
Bots have been around since the internet was invented. The most famous bots are those that search the internet, to find quality websites like this one, and then you have stock market scalping bots, automated shopping bots, chatbots, and the more infamous ones are the GPUs scalping bots! And probably why you are here.
Beating the GPU Scalpers
We have all been trying to buy the latest Nvidia GPUs like the RTX 3060, RTX 3070, 3080, and RTX 3090 for those who can afford them. Also, the most popular AMD CPUs are pretty hard to buy. This is all because of the general shortage of silicon, which is affecting, not only computing but also the whole automotive industry, and other sectors.
Also, the greedy scalpers are not helping at all. The very few GPUs available on the market are automatically purchased by ultra-fast bots, probably written in Python, that are capable of solving captchas with AI, and let’s be honest, better than we can do.
Crypto Miners are scalping too
The demand is there because of crypto mining as well. Bitcoin is skyrocketing in value and more and more people are buying GPUs, not for gaming, not for Deep Learning, but for mining Bitcoin! And let’s face it, the miners will have a lot more resources than a “simple” scalper who is trying to sell for a profit a GPU on eBay.
Why create your own Python Bot
The most important reason for creating your own python bot is that “knowledge is power”. By being able to make your own Python bot the possibilities are endless.
Not only you will be able to beat the GPU scalpers and get that nearly impossible to buy GPU, but you can also collect all kinds of useful data from the internet with Data Scraping, which is extremely powerful and useful for a variety of fields that feed on data(e.g. machine learning).
You can also use bots to deliver useful services for the internet too. For example, a few years ago when I was trying to pass my driving license, I couldn’t book my driving test without waiting 3 months for an available slot because there was huge demand for driving tests and not too many slots available. But then sometimes someone would cancel their driving test at the last minute, opening up an available slot. But there was no way for me to know, without being on the website 24/7.
I then found a website that would scalp the slots for me, for a small fee. And voila, after I failed my driving test(UK — not USA), I was able to book my driving test again for the following week.
Whoever created that service, made a huge profit and at the same were able to provide useful service using an “ethical” bot.
You don’t know how to code in Python
If you don’t know how to code or you don’t know Python, I recommend you to learn it. Python is an extremely popular language applicable to a lot of fields. You can’t go wrong with it. And it turns out many bots on the internet are written in Python, so you must learn Python!
There are plenty of Python courses available on the internet. My recommendation is that you take one basic Python course to get started, in order to learn the basics of the language. There are so many good courses for Python. Probably the most popular is a course by Dr. Chuch Severance from the University of Michigan available on Coursera. If I was starting to learn Python today, he would be my first stop:
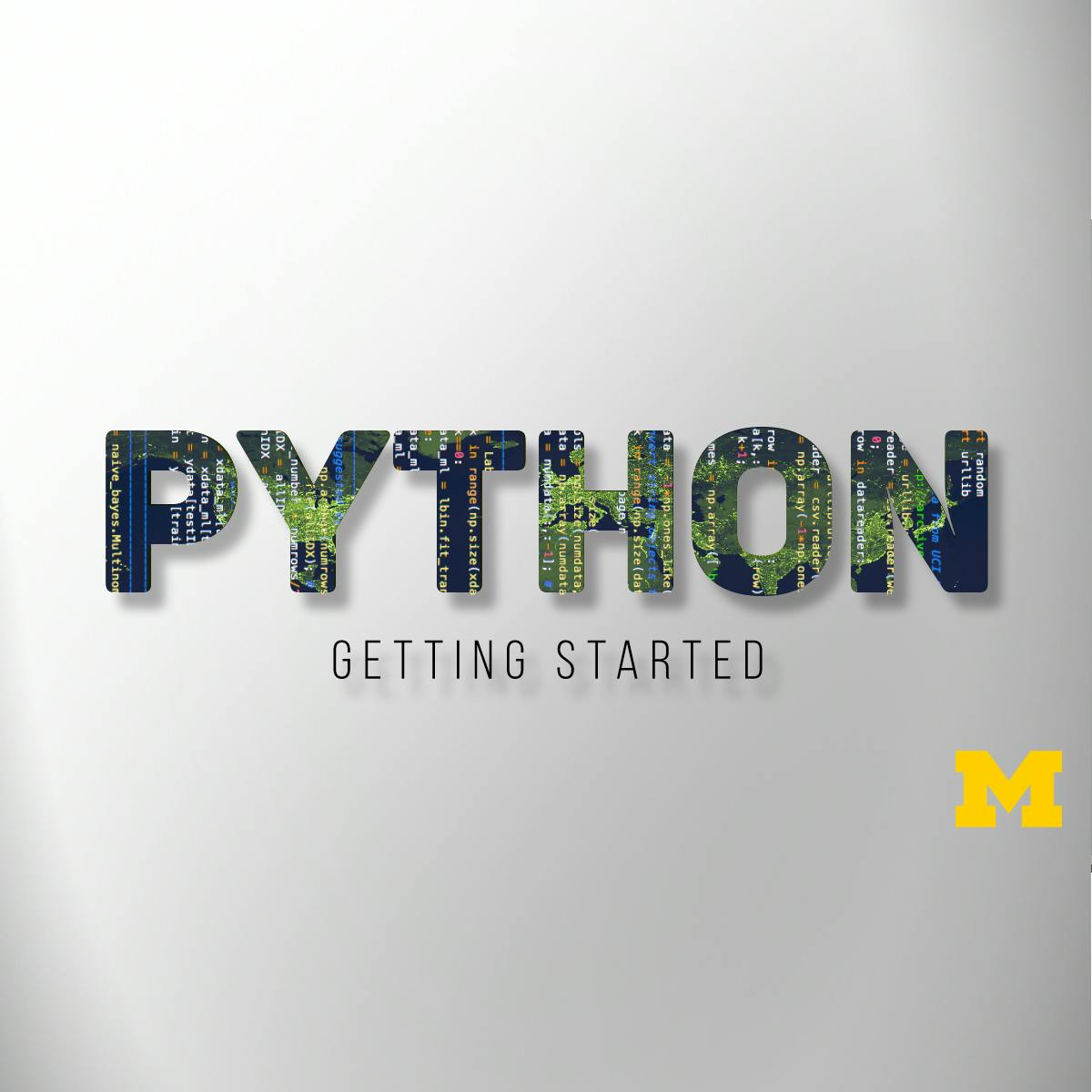
Programming for Everybody (Getting Started with Python)
Don’t get discouraged by the 13h in the course, every single minute will be worth it. And definitely don’t waste more time on other courses. You just need one course to learn Python. Nothing more.
Once you can code in Python, most of the learning will be done by doing.
How to create a simple Python bot
Enough of talking. Let’s create a simple Python bot, which you can use as your starting point. This python bot will check for stock of the latest RTX series from the newegg.com website as an example.
Installing Python
Before we do any Python Development, you need to somehow install Python on your computer. There are several methods on how to install Python.
Installing Standalone Python
You can install Python directly by downloading the correct installer for your OS (Windows or Mac) from python.org and installing it. Please see the instructions on how to do that here.
Installing Python with Anaconda
Even though it is perfectly acceptable to install Python standalone, many choose to install Python with Anaconda. And why is that?
Why Anaconda?
Anaconda is a toolkit that includes Python and libraries that are are the favorites for most Data Science, Machine learning, web scraping projects on the web. And it already includes Jupyter Lab, a web-based IDE, very useful for the development of our Python Bot.
With Anaconda, we can run different versions of Python in isolated environments which only contain the Python libraries and versions that we need. While still having access to pip we also have access to the conda package manager which allows us to install pre-compiled Python libraries. Contrast this to pip which instead of providing the compiled binary of a library, needs to compile any library from wheels during installation.
How to Install Anaconda
It is possible to install Anaconda in Windows, MacOS, or Linux. We will install Anaconda in Windows 10 for this tutorial. Before starting make sure that you have at least 3GB of disk space available.
Downloading the Installer
First, let’s download the installer from the Anaconda website.
Starting the installation

At this point, you have a choice to install for yourself or for “All Users”. Just go with the recommended option, and install it just for yourself in your own userspace.
Adding Anaconda to Windows PATH environment variable
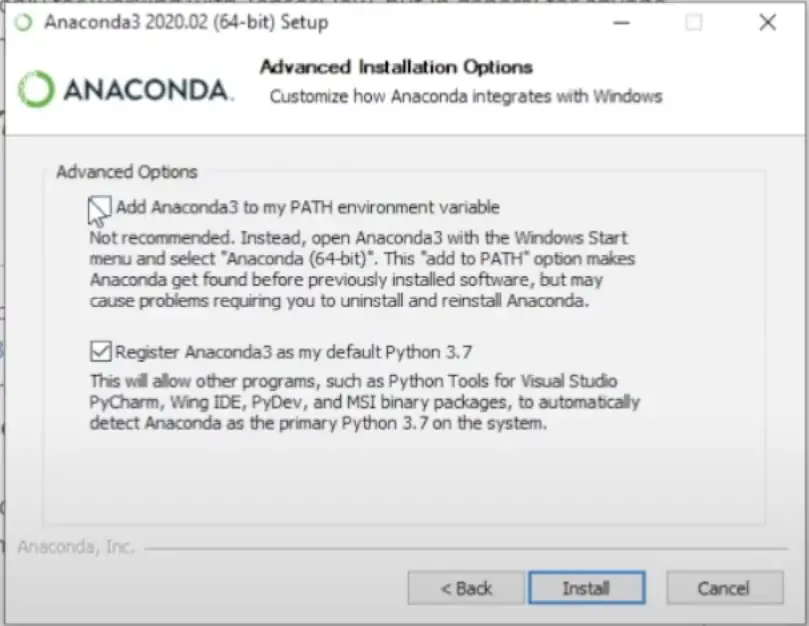
At this stage, you will want to tick the checkbox “Add Anaconda3 to my PATH variable”. This will require Administration privileges.
You will notice a warning, which is highlighted in red, once you tick the box. If you are the main user of your computer and you don’t have any previously installed versions of Anaconda or Python, then you shouldn’t have any issues. In the worst-case scenario, it is always possible to delete the variable from your PATH at a later stage.

And finally, the installation completes:
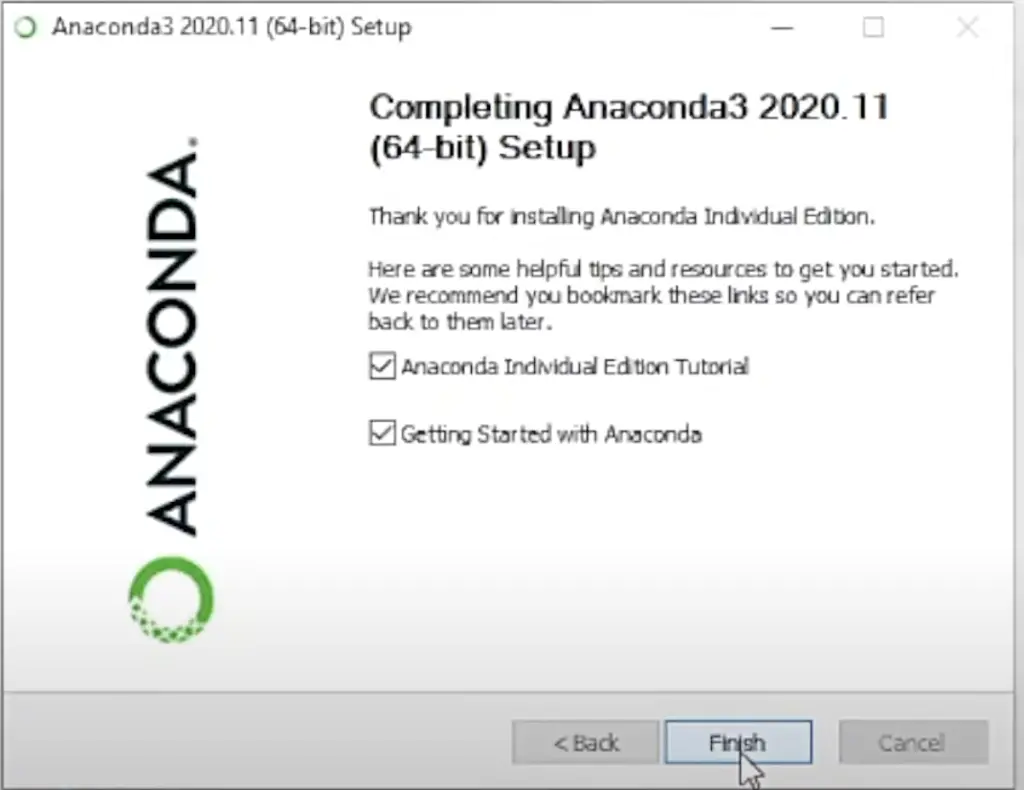
Starting Anaconda Navigator
To get started we open the Anaconda Navigator:
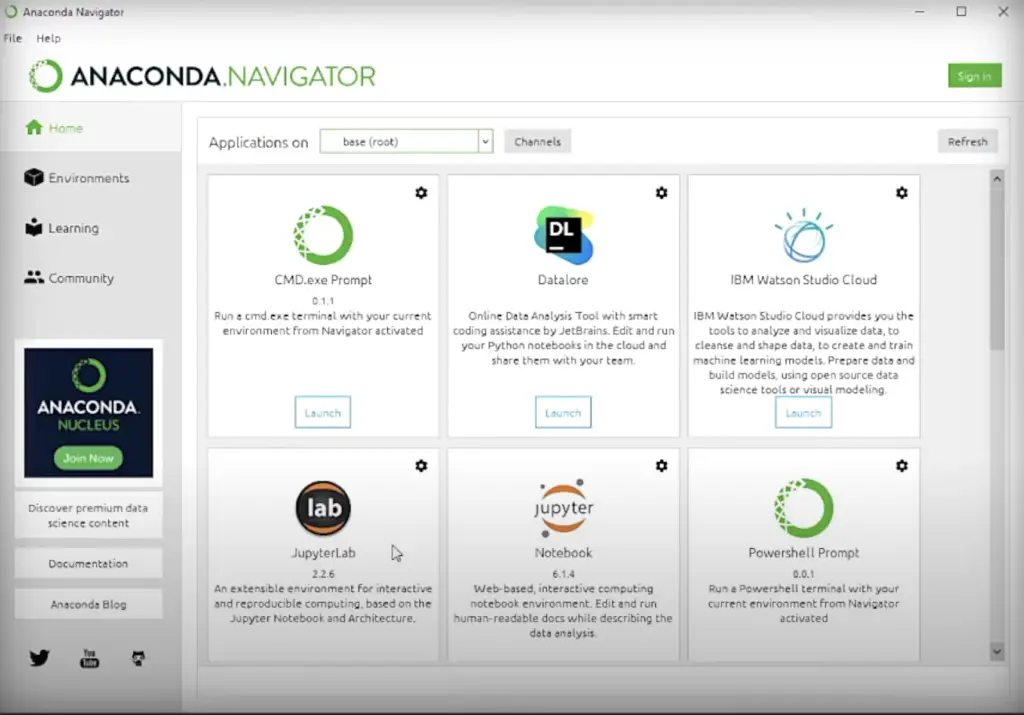
Creating an environment in Anaconda
To create a new Anaconda Environment, click on Environments:

And here we can type gpu_python_bot in the environment name. One of the advantages of Anaconda is that we can have multiple environments using different versions of Pythons. But in our case Python 3.8 is ok. So we can leave the default value.
Installing the required libraries
To follow the tutorial we need to install the following libraries:
- selenium
- python-chromedriver-binary
- bs4 ( Beautiful Soup)
- pandas
Let’s open a command prompt and activate the newly created environment:
$ conda activate gpu_python_bot
> (gpu_python_bot)....
Now we can install the required libraries:
conda install selenium
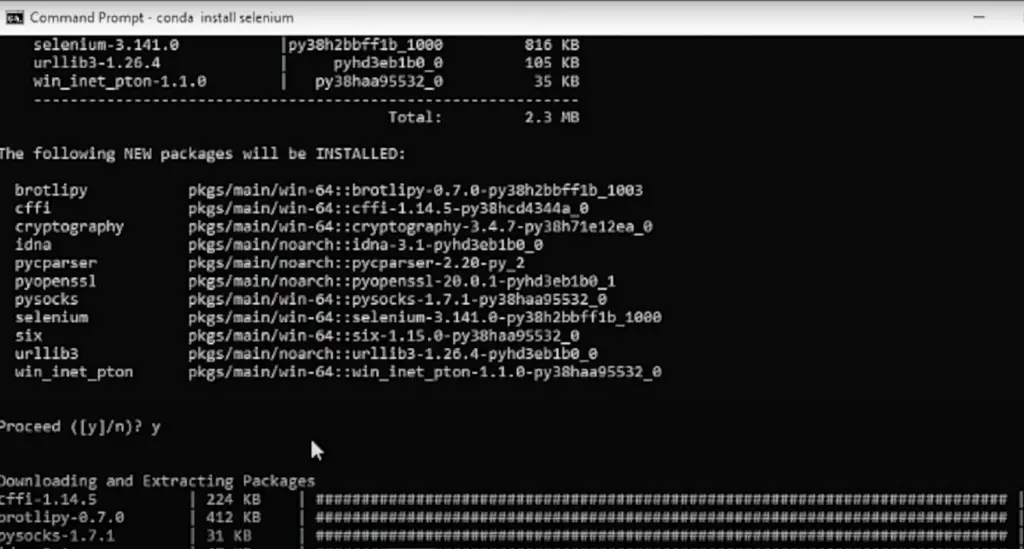
How to install the Python Chromedriver Binary
If you try to install the python-chromedriver-binary with:
conda install python-chromedriver-binary
It will most likely fail with this error message:

What the error we saw means, is that conda was not able to find the python-chromedriver-binary in the default channel.
For this reason, we have to look for this python library from an alternative source. In our case the alternative source is the conda-forge, a community-led collection of distributions for the conda package manager.
Let’s try to install again, but this time providing the conda-forge channel.
$ conda install -c conda-forge python-chromedriver-binary
>...
How to install The Pandas and BeautifulSoup Library
We install Pandas and BeautifulSoup as we are likely to need them:
$ conda install pandas
...
$ conda install bs4
...
Resources – Web Scraping Videos + Source Code
Source Code: https://github.com/armindocachada/yeelight-nvidia-rtx-ryzen-stock-checker
Source Code: https://gist.github.com/armindocachada/e54309743734e091a7b849d66c5fc390
Source Code: https://github.com/armindocachada/yeelight-nvidia-rtx-ryzen-stock-checker/blob/main/Buy%20Bot%20-%20RTX%20GPU.ipynb
Source Code: Amazon Price Scraper with WhatsApp Notifications (github.com)
While trying to create a Python Bot you might face a variety of errors, watch this video: